State design pattern provides a mechanism to change behavior of an object based on the object’s state. We can see lot of real world examples for the need of state design pattern. Think of our kitchen mixer, which has a step down motor inside and a control interface. Using that knob we can increase / decrease the speed of the mixer. Based on speed state the behavior changes.
Class Diagram for State Design Pattern
Let us take an example scenario using a mobile. With respect to alerts, a mobile can be in different states. For example, vibration and silent. Based on this alert state, behavior of the mobile changes when an alert is to be done. Which is a suitable scenario for state design pattern. Following class diagram is for this example scenario,
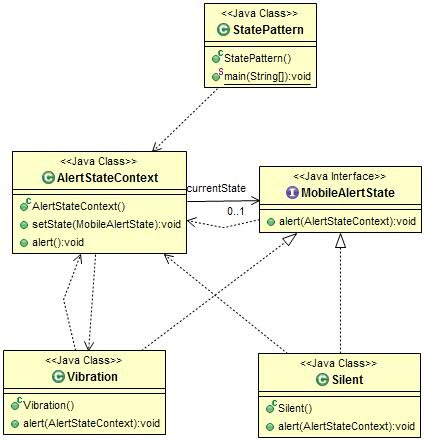
State Design Pattern Java Example
MobileAlertState.java
This interface represents the different states involved. All the states should implement this interface.
package com.javapapers.designpatterns.state; public interface MobileAlertState { public void alert(AlertStateContext ctx); } |
AlertStateContext.java
This class maintains the current state and is the core of the state design pattern. A client should access / run the whole setup through this class.
package com.javapapers.designpatterns.state; public class AlertStateContext { private MobileAlertState currentState; public AlertStateContext() { currentState = new Vibration(); } public void setState(MobileAlertState state) { currentState = state; } public void alert() { currentState.alert( this ); } } |
Vibration.java
Representation of a state implementing the abstract state object.
package com.javapapers.designpatterns.state; public class Vibration implements MobileAlertState { @Override public void alert(AlertStateContext ctx) { System.out.println("vibration..."); } } |
Silent.java
Representation of a state implementing the abstract state object.
package com.javapapers.designpatterns.state; public class Silent implements MobileAlertState { @Override public void alert(AlertStateContext ctx) { System.out.println("silent..."); } } |
StatePattern.java
package com.javapapers.designpatterns.state; public class StatePattern { public static void main(String[] args) { AlertStateContext stateContext = new AlertStateContext(); stateContext.alert(); stateContext.alert(); stateContext.setState( new Silent()); stateContext.alert(); stateContext.alert(); stateContext.alert(); } } |
Example State Pattern Code Output
vibration... vibration... silent... silent... silent...
It is a well executed post. I like the diagram most. It is a helpful informative post. Thanks for sharing this great information.
ReplyDeleteMy Signature: Top 5 Medical Websites for Your Medical Clinic.